Implement Facebook-style comment suggestions using Gemini API
As someone who enjoys experimenting with design and technology, I love finding ways to enhance user experiences. Previously, I implemented a comment suggestion feature on my website, inspired by Facebook’s smart replies. While it was fun and users enjoyed using it, the comments were static, randomly pulled from predefined positive and negative arrays. Over time, they started to feel repetitive and spam-like.
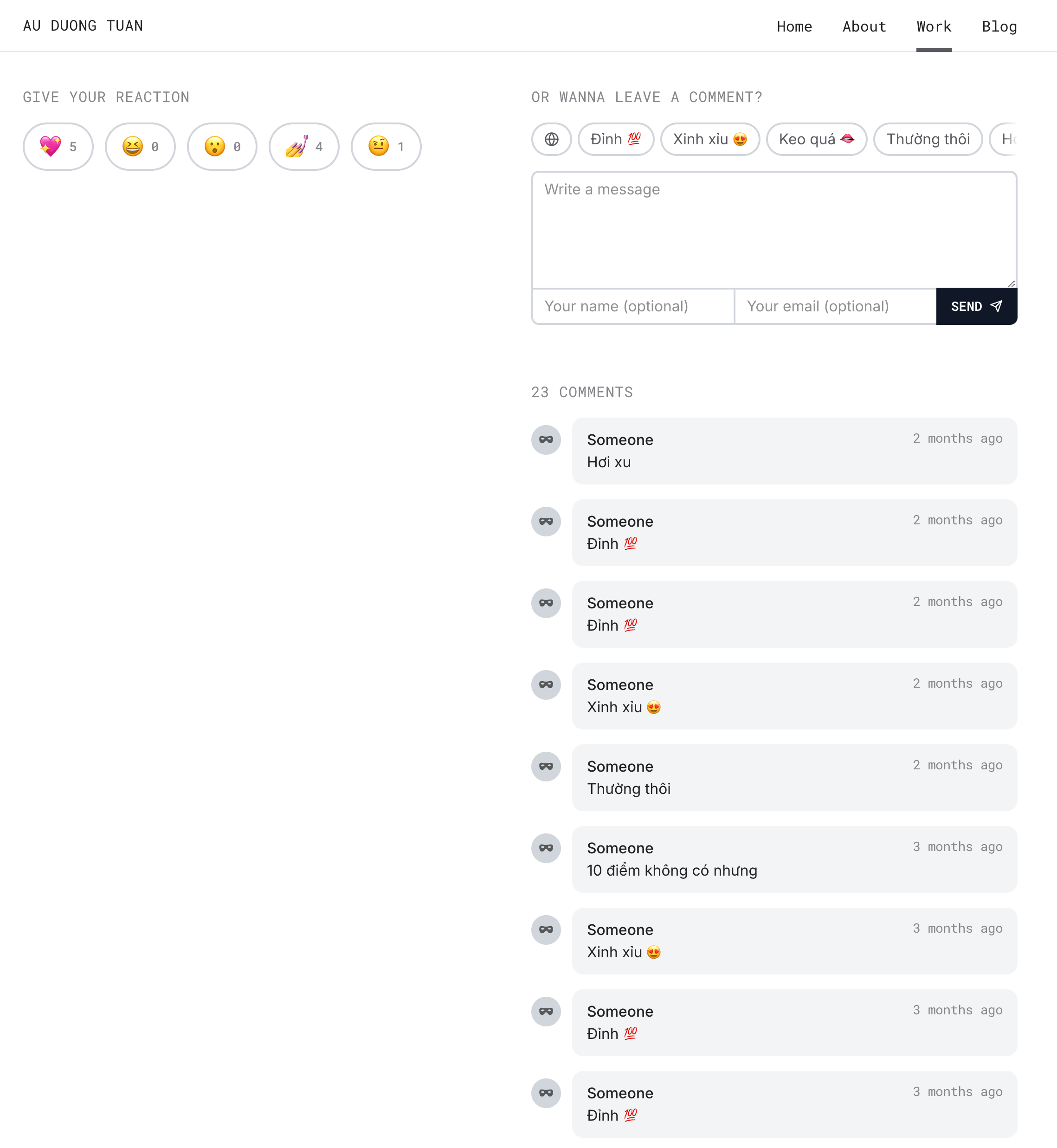
The Upgrade: AI-Generated Contextual Comments
This weekend, I decided to level up this feature using AI. Instead of static responses, I integrated the Gemini API to dynamically generate contextually relevant comment suggestions based on each blog post's content. Now, every set of suggestions feels fresh, engaging, and aligned with the article it appears under.
The Challenge: Crafting the Perfect Prompt
Getting AI to generate the right kind of comments wasn’t straightforward. I needed the suggestions to be:
- Short and concise (limited to 10 words).
- Fun and engaging, with a friendly tone.
- Diverse in sentiment, ensuring that some comments reflect constructive feedback.
- Language-specific, incorporating trendy phrases in both English and Vietnamese.
- Context-aware, so the comments match the article's subject.
After several iterations, I refined the following prompt:
const prompt = `Read this article and give me 3 sets of comments in Vietnamese and English, each set containing 7 suggested comments like the way Facebook does.
Try to write them concisely, omitting redundant words, and limiting them to 10 words.
Ensure the comments are specific and relevant to the content of the article.
Write with a fun, friendly voice, and add different emojis to each comment.
Include two slightly negative comments, but keep them lighthearted.
For Vietnamese, use trendy words like "tái châu," "keo," "đỉnh nóc," "xịn vậy má" for positive comments and "xu cà na" for negative ones—but sparingly.
For English, mix in trendy slang like "slay" or "stan" occasionally.
One comment should contain only one trendy word.
Return in JSON format, structured as follows:
{
vietnamese: [["comment 1", "comment 2"], ["comment 3", "comment 4"]],
english: [["comment 1", "comment 2"], ["comment 3", "comment 4"]]
}
${url}`;
The Implementation
With the prompt refined, I add an API route to fetch suggestions dynamically to my Next.js website. The server-side function:
- Retrieves the page URL.
- Calls the Gemini API with the prompt.
- Caches the response for 31 days to optimize performance.
- Returns the AI-generated comments in JSON format.
Using the Gemini Node SDK (@google/generative-ai)
To integrate the Gemini API, I used the official Node.js SDK, @google/generative-ai
. Here’s how to set it up:
- Install the SDK:
pnpm install @google/generative-ai
- Import and configure the Gemini model:
import { GoogleGenerativeAI } from "@google/generative-ai"; const genAI = new GoogleGenerativeAI(process.env.GEMINI_API_KEY || ""); const model = genAI.getGenerativeModel({ model: "gemini-2.0-flash-exp" });
- Fetch AI-generated comment suggestions:
export async function getCommentSuggestion(url: string) { const prompt = `...`; // (Prompt as described above) const result = await model.generateContent(prompt); return JSON.parse(result.response.text()); }
The API Endpoint
The API endpoint handles requests efficiently:
import { NextApiRequest, NextApiResponse } from "next";
import { getCommentSuggestion } from "@lib/commentSuggestion";
import cacheData from "memory-cache";
const commentSuggestionAPI = async (req: NextApiRequest, res: NextApiResponse) => {
if (req.method === "GET") {
const page = req.query.page as string;
if (!page.startsWith(process.env.NEXT_PUBLIC_PRODUCTION_WEB_URL as string)) {
return res.status(400).json({ error: "Invalid page URL" });
}
const id = "comment-suggestion_" + page;
let data = cacheData.get(id);
if (!data) {
data = await getCommentSuggestion(page);
cacheData.put(id, data, 31 * 24 * 1000 * 60 * 60);
}
return res.status(200).json(data);
}
};
export default commentSuggestionAPI;
The Results
With this upgrade, the comments feel more natural, engaging, and interactive. Users are responding positively to the AI-generated suggestions, and the experience is far more dynamic than before.
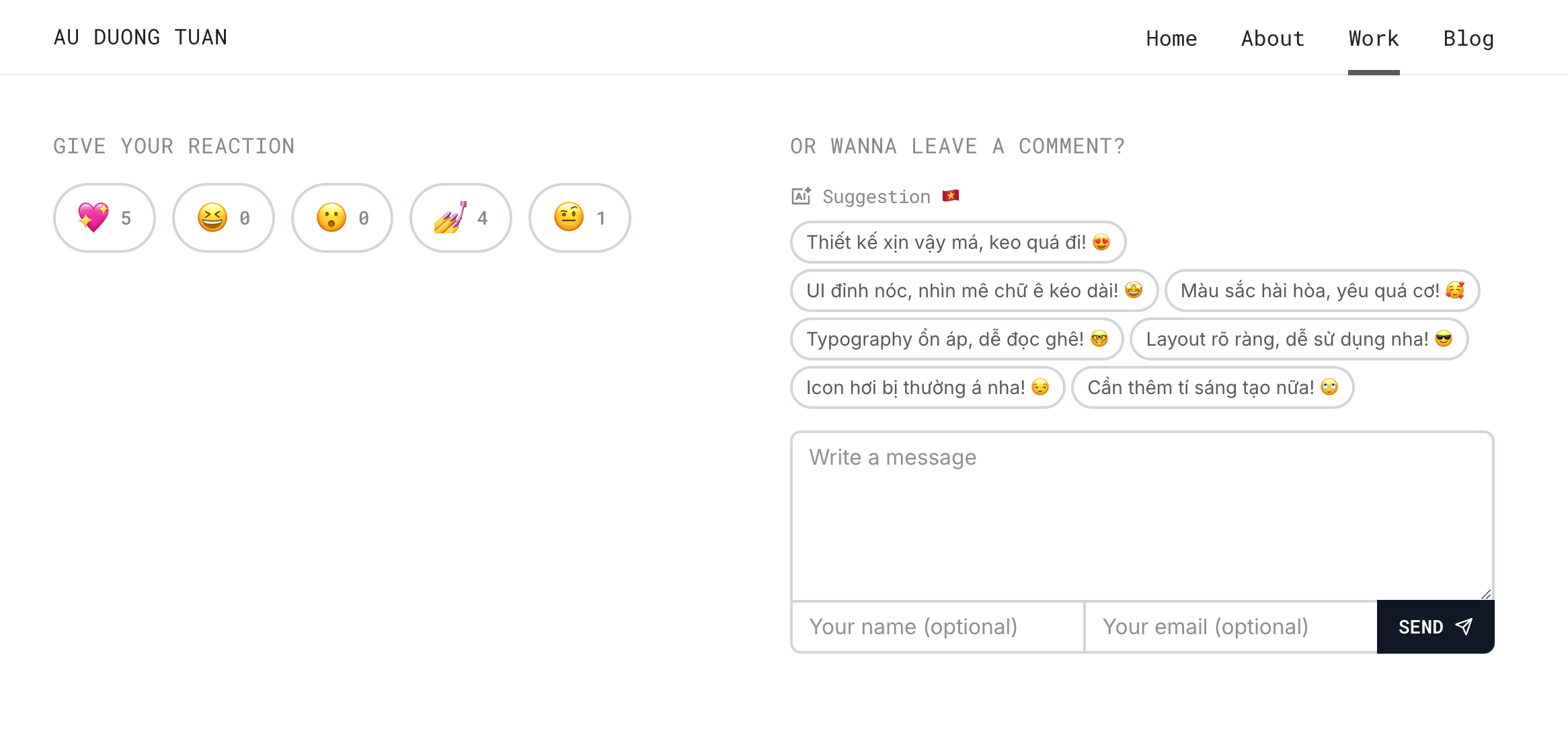
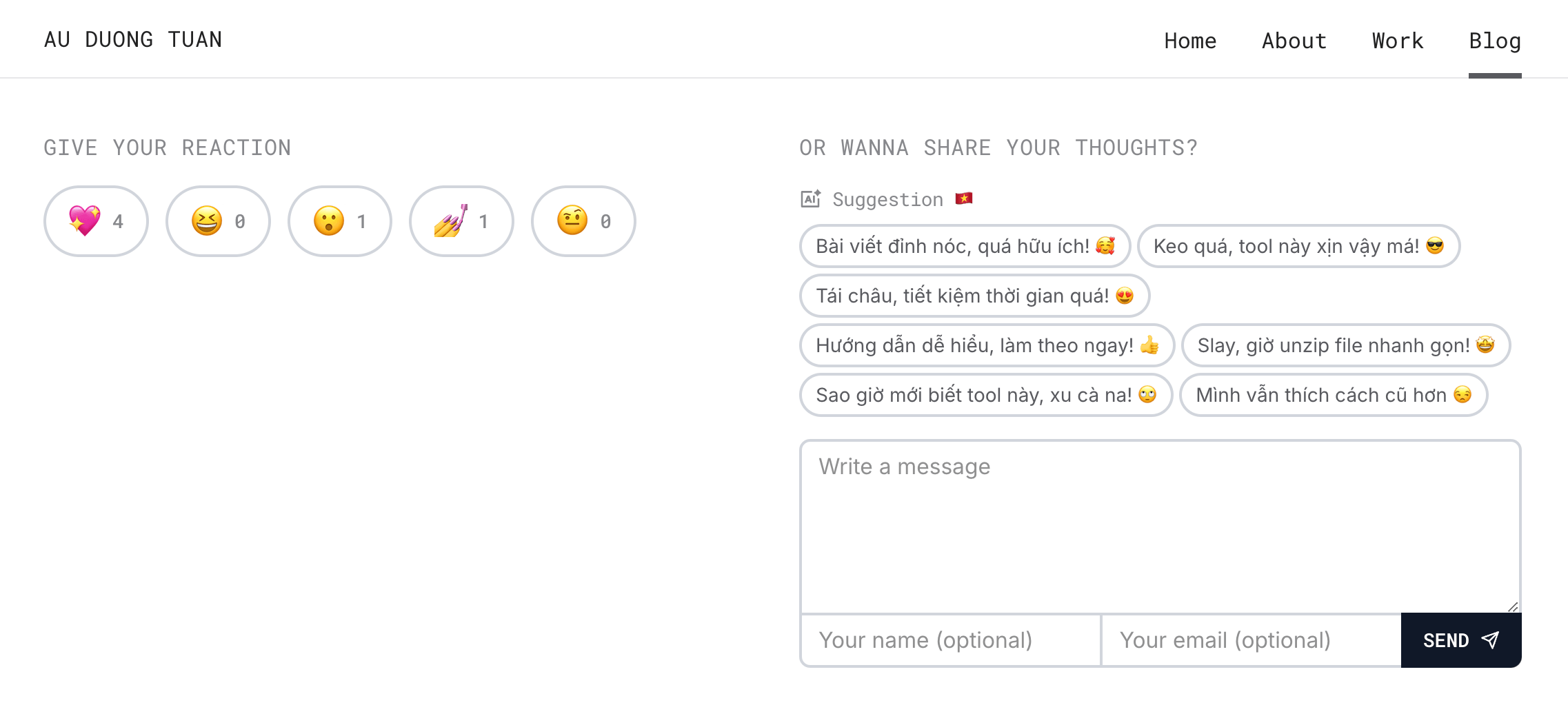
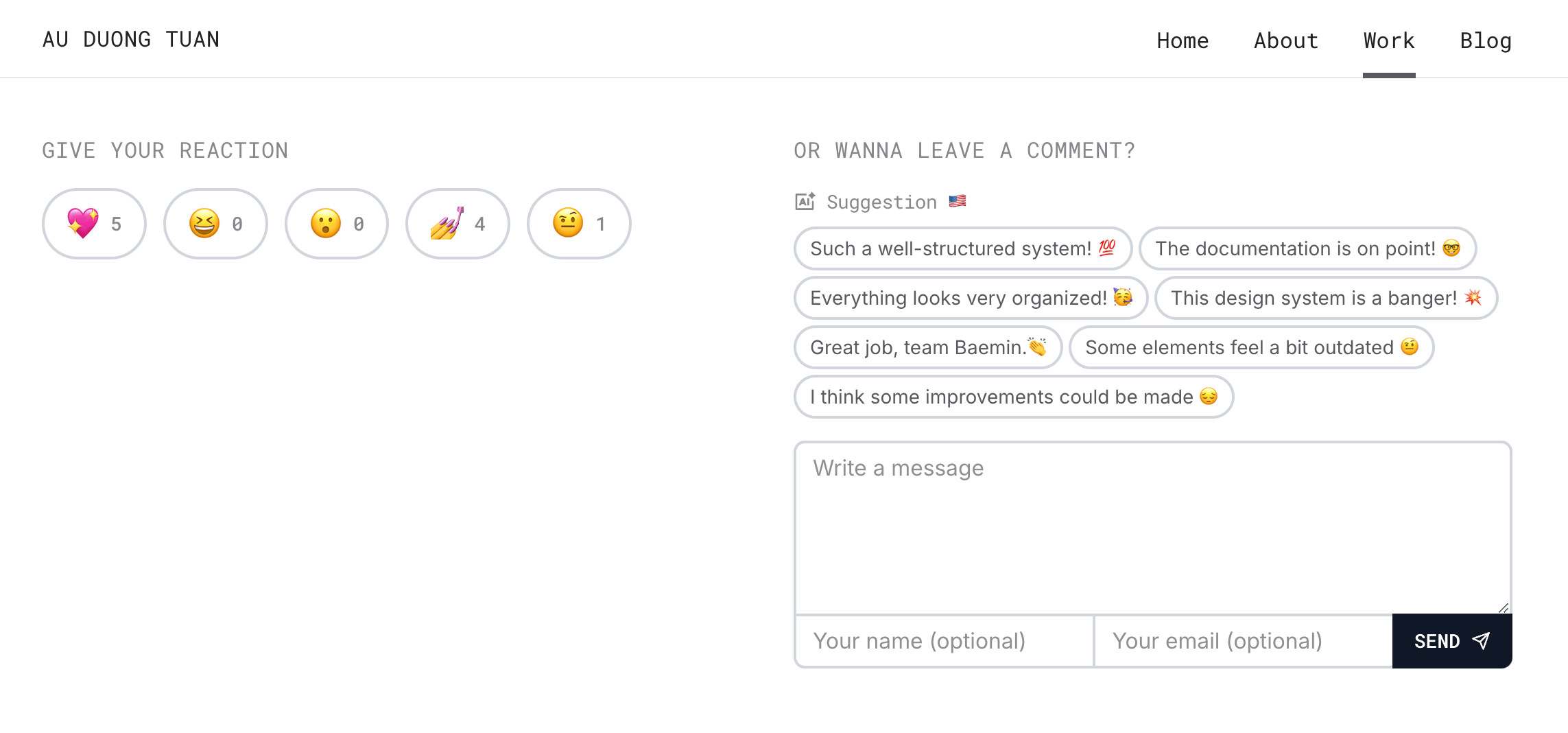
This project was a great exercise in prompt engineering and AI-powered UX improvements, making user interactions feel more intuitive and engaging. Excited to keep refining it further!
Give your reaction
Or wanna share your thoughts?
Suggestion